express + ts 创建服务端 + 打包成为 exe
express + ts 创建服务端 + 打包成为 exe
初始化 项目
npm init
or
yarn init
or
pnpm init
安装 express 依赖
npm install express --save
or
yarn add express
or
pnpm install express
安装 typescript 依赖
pnpm install typescript ts-node-dev @types/express @types/node ts-node
or
yarn add typescript ts-node-dev @types/express @types/node ts-node
or
npm install typescript ts-node-dev @types/express @types/node ts-node
安装 morgan logger body-parser cookie-parser 依赖
pnpm install morgan logger body-parser cookie-parser -S
or
yarn add morgan logger body-parser cookie-parser -S
or
npm install morgan logger body-parser cookie-parser -S
创建 tsconfig.json 文件
{
"compilerOptions": {
"module": "commonjs",
"esModuleInterop": true,
"target": "es6",
"rootDir": "./",
"outDir": "./build",
"strict": true
}
}
创建 app.ts 文件
import express from "express";
import path from "path";
var logger = require("morgan");
var cookieParser = require("cookie-parser");
var bodyParser = require("body-parser");
const app = express();
/** 使用 日志中间件 */
app.use(logger("dev"));
/** 将 json 数据解析到 req.body */
app.use(express.json());
/** 将 url-encoded 数据解析到 req.body */
app.use(express.urlencoded({ extended: true }));
/**
* 设置post最大值
* post 最大值为 100MB
* */
app.use(bodyParser.json({ limit: "100MB" }));
app.use(bodyParser.urlencoded({ limit: "100MB", extended: false }));
/**
* 设置 cookieParser
* */
app.use(cookieParser());
/** 处理 404 */
app.use(function (req: any, res: any, next: any) {
return res.send({
code: 404,
message: "404 not found",
});
});
/** 处理错误 */
app.use(function (err: any, req: any, res: any, next: any) {
res.locals.message = err.message;
res.locals.error = req.app.get("env") === "development" ? err : {};
res.status(err.status || 500);
res.render("error");
});
export default app;
新建 public 、 upload 、 routes 、 views 、jest 、config 、 tool 文件夹
public 公共资源文件夹
upload 上传文件文件夹
routes 路由文件夹
views 视图文件夹
jest 测试文件夹
config 配置文件夹
tool 工具文件夹
# windows
mkdir public upload routes views jest config tool
# linux
mkdir -p public upload routes views jest config tool
# mac
mkdir -p public upload routes views jest config tool
在 app.ts 文件中引入 引入静态文件配置
/**静态文件配置*/
app.use(express.static(path.join(__dirname, "public")));
app.use(express.static(path.join(__dirname, "upload")));
app.use(express.static(path.join(__dirname, "views")));
创建 config/config.ts 配置文件
/**基础配置*/
export const config = {
/**端口*/
port: "3005",
/**静态资源目录*/
staticDir: ["public", "uploads"],
/**是否为开发环境*/
development: true,
/**最大上传文件大小*/
maxPostSize: "100mb",
/**上传文件目录*/
uploadDir: "uploads",
/**是否开启跨域*/
crosEnable: true,
/**跨域配置*/
cors: {
origin: "*",
methods: "GET,HEAD,PUT,PATCH,POST,DELETE",
allowedHeaders: "Content-Type, Authorization, X-Requested-With",
credentials: "true",
maxAge: "86400",
},
/**http图片地址*/
httpImgUrl: "",
/**http文件地址*/
httpFileUrl: "",
/**jwt密钥*/
secretOrPrivateKey: "blog",
/**jwt过期时间*/
expiresIn: 60 * 60 * 24 * 1,
/**当前可以输出的日志级别*/
logLevel: {
info: true,
warn: true,
error: true,
debug: true,
success: true,
log: true,
},
};
/** 数据库基础配置*/
export const sqlConfig = {
host: "", //这是数据库的地址
user: "", //需要用户的名字
password: "", //用户密码 ,如果你没有密码,直接双引号就是
database: "", //数据库名字
};
/** redis 配置*/
export const redisConfig = {
host: "",
port: "",
password: "",
};
/**错误代码*/
export const errorCode = {
/** 401 无权限*/
401: {
code: 401,
message: "无权限",
},
/** 404 找不到数据*/
404: {
code: 404,
message: "找不到数据",
},
/** 500 服务器错误*/
500: {
code: 500,
message: "服务器错误",
},
};
/**成功代码*/
export const successCode = {
200: {
code: 200,
message: "成功",
},
};
在 app.ts 文件中引入 config/config.ts 文件 并修改配置
import { config, errorCode } from "./config/config";
/**
* 设置post最大值
* post 最大值为 100MB
* */
app.use(bodyParser.json({ limit: config.maxPostSize }));
app.use(bodyParser.urlencoded({ limit: config.maxPostSize, extended: false }));
/**
* 设置跨域
* */
if (config.crosEnable) {
app.all(config.cors.origin, async (req, res, next) => {
res.header("Access-Control-Allow-Origin", req.headers.origin); //需要显示设置来源,不能使用‘*’
res.header(
"Access-Control-Allow-Headers",
config.cors?.allowedHeaders ||
"Origin, X-Requested-With, Content-Type, Accept"
);
res.header(
"Access-Control-Allow-Methods",
config.cors?.methods || "PUT,POST,GET,DELETE,OPTIONS"
);
res.header(
"Access-Control-Allow-Credentials",
config.cors.credentials || "true"
); //需要加上这个
res.header("Access-Control-Max-Age", config.cors.maxAge || "86400");
next();
});
}
/** 处理 404 */
app.use(function (req: any, res: any, next: any) {
return res.send({
...errorCode[404],
});
});
创建 routes/index.ts 文件
import express from "express";
const router = express.Router();
router.get("/", function (req, res, next) {
res.send("hello world");
});
export default router;
在 app.ts 文件中引入 routes/index.ts 文件
/**引入路由*/
import indexRouter from "./routes/index";
/**设置路由*/
app.use("/", Router);
安装 debug 依赖
npm install debug -S
or
pnpm install debug -S
or
yarn add debug -S
创建 bin/www.ts 文件
import app from "../app";
import http from "http";
import { config } from "../config/config";
var debug = require("debug")("serve-blog:server");
var port = normalizePort(config.port || "3005");
app.set("port", port);
console.log(`Server is running on port ${port} `);
/**
* 创建 HTTP server
*/
var server = http.createServer(app);
/**
* 监听 HTTP server 的错误和监听事件
* 监听 HTTP server 端口
*/
server.listen(port);
server.on("error", onError);
server.on("listening", onListening);
/**
* 将端口标准化为数字、字符串或false
*/
function normalizePort(val: string) {
var port = parseInt(val, 10);
if (isNaN(port)) {
// named pipe
return val;
}
if (port >= 0) {
// port number
return port;
}
return false;
}
/**
* HTTP服务器“错误”事件的事件侦听器。
*/
function onError(error: any) {
if (error.syscall !== "listen") {
throw error;
}
var bind = typeof port === "string" ? "Pipe " + port : "Port " + port;
// handle specific listen errors with friendly messages
switch (error.code) {
case "EACCES":
console.error(bind + " requires elevated privileges");
process.exit(1);
break;
case "EADDRINUSE":
console.error(bind + " is already in use");
process.exit(1);
break;
default:
throw error;
}
}
/**
* HTTP服务器“侦听”事件的事件侦听器。
*/
function onListening() {
var addr = server.address();
if (addr) {
var bind =
typeof addr === "string" ? "pipe " + addr : "port " + addr.port;
debug("Listening on " + bind);
}
}
安装 nodemon 监听文件修改
npm install nodemon -S
or
pnpm install nodemon -S
or
yarn add nodemon -S
在 package.json 文件中添加 scripts 配置
"scripts": {
"dev": "nodemon bin/www",
"build" : "sc -p tsconfig.json",
"start": "nodemon build/bin/www"
}
在 package.json 文件中添加 入口文件
"main": "app.ts",
运行项目
npm run dev
or
pnpm run dev
or
yarn run dev
打包项目成为命令行工具
安装 PKG
npm install pkg -S
or
pnpm install pkg -S
or
yarn add pkg -S
在 package.json 文件中添加 scripts 配置
"pkg": {
"scripts": "build/**/*.js",
"assets": "public/**/*",
"targets": [
"node18-win-x64",
"node18-linux-x64",
"node18-macos-x64"
]
},
安装 npm-run-all 同时运行多个命令
npm install npm-run-all -S
or
pnpm install npm-run-all -S
or
yarn add npm-run-all -S
在 package.json 文件中添加 scripts 配置
"scripts": {
"prepare:exe": "run-s prebuild:exe",
"prebuild:exe": "if not exist build\\exe mkdir build\\exe",
"build:exe": "run-s prepare:exe pkg:exe",
"build:exe-linux": "run-s prepare:exe pkg:exe-linux",
"prebuild:exe-mac": "run-s prepare:exe pkg:exe-mac",
"pkg:exe": "pkg build/bin/www.js --targets node18-win-x64 --output build/exe/blog-admin.exe",
"pkg:exe-linux": "pkg build/bin/www.js --targets node18-linux-x64 --output build/exe/blog-admin-linux",
"pkg:exe-mac": "pkg build/bin/www.js --targets node18-macos-x64 --output build/exe/blog-admin-macos.app"
}
打包项目
npm run build
or
pnpm run build
or
yarn run build
打包项目为指定文件 这里打包为 exe 文件
npm run build:exe
or
pnpm run build:exe
or
yarn run build:exe
测试的命令
安装 jest ts-jest @types/jest 依赖 开发环境
npm install jest ts-jest @types/jest -D
or
pnpm install jest ts-jest @types/jest -D
or
yarn add jest ts-jest @types/jest -D
创建 jest/jest.config.ts 文件
module.exports = {
preset: 'ts-jest',
testEnvironment: 'node',
testMatch: [
"**/__tests__/**/*.+(ts|tsx|js)",
"**/?(*.)+(spec|test).+(ts|tsx|js)",
'<rootDir>/**/*.test.ts'
],
rootDir : '../',
};
配置 package.json 文件
"scripts": {
"test": "jest --config jest/jest.config.js"
}
生成一个建议的工具类 tool/sum.ts
export default function sum(a: number, b: number): number {
return a + b;
}
生成一个测试类 jest/sum.test.ts
import sum from '../tool/sum';
describe('sum module', () => {
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
})
test('adds 1 + 2 not to equal 4', () => {
expect(sum(1, 2)).not.toBe(4);
})
})
开始测试
npm run test
or
pnpm run test
or
yarn run test
指定单个测试文件
npm run test -- --testPathPattern=sum.test.ts
or
pnpm run test -- --testPathPattern=sum.test.ts
or
yarn run test -- --testPathPattern=sum.test.ts
生成一个测试类 jest/indexRouter.test.ts
import request from 'request';
describe('indexRouter', () => {
test('GET /', (done) => {
request.get('http://localhost:3005', (error, response, body) => {
expect(response.statusCode).toBe(200);
expect(body).toBe('hello world');
})
})
})
一个小前端
我是一个小前端
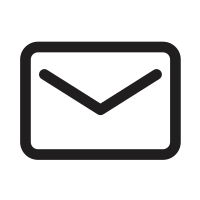
zs.duan@qq.com
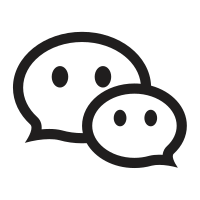
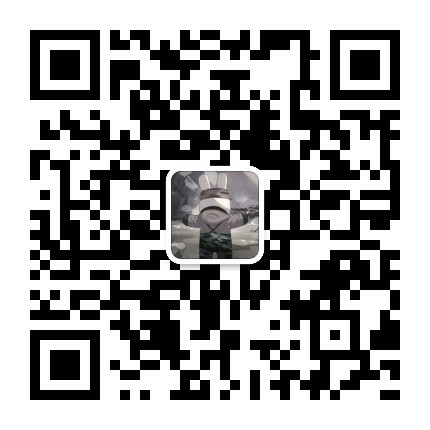
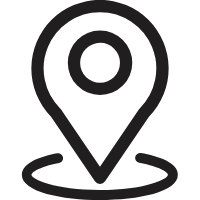
重庆市沙坪坝
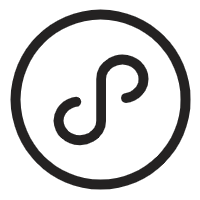
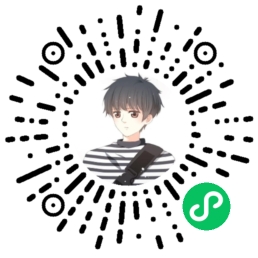
我的标签
小程序
harmonyOS
HTML
微信小程序
javaSrcipt
typeSrcipt
vue
uniapp
nodejs
react
防篡改
nginx
mysql
请求加解密
还没有人评论 快来占位置吧